Introduction
BASE URL
https://api.employmenthero.com
CLIENT LIBRARIES
By default, the Employment Hero API Docs demonstrate using curl to interact with the API over HTTP.
The Employment Hero API is a RESTful-based API that returns JSON-encoded responses and uses standard HTTP response codes, authentication, and verbs.
Once connected, you'll have access to valuable HR data which can then be leveraged within your app or integration to power workflows and insights. In order to test the Employment Hero API, we recommend using a Bearer token for authentication - see this section for more information.
Authentication
The preferred secure way to authenticate against and access the Employment Hero API is by using an OAuth 2.0 protocol. Our OAuth 2.0 process described below follows a basic pattern from OAuth 2.0 documentation, please follow the steps below at a high level.
Basic steps
- To begin, register your application with the Employment Hero platform through our Developer Portal.
- Obtain your client credentials including client ID and secret from the registration page. They will be required in the upcoming steps.
- Authorise your client application with the Employment Hero through our Authorisation Server. You will be redirected to Employment Hero login page if you have not yet logged in. For a successful login, a confirmation page will be shown to acquire user scopes provision.
- Successful authorisation results in a redirection to your provided redirect uri(s) with the authorisation code included.
- Use the authorisation code to request an access token. The response will include both the access and refresh tokens. Extract the access token from the response, and send the access token to the Employment Hero API that you wish to access.
- Please note that for security purposes, the lifetime of the access token is 15 minutes. You can obtain another access token by sending a refresh token request to the Employment Hero Authorisation Server. When refreshing the access token, the new refresh token will be returned as well and does not currently have an expiration time. Clients applications should be built to always store the returned refresh token. Please be sure to keep the refresh token safe and secure.
Developer Portal Access
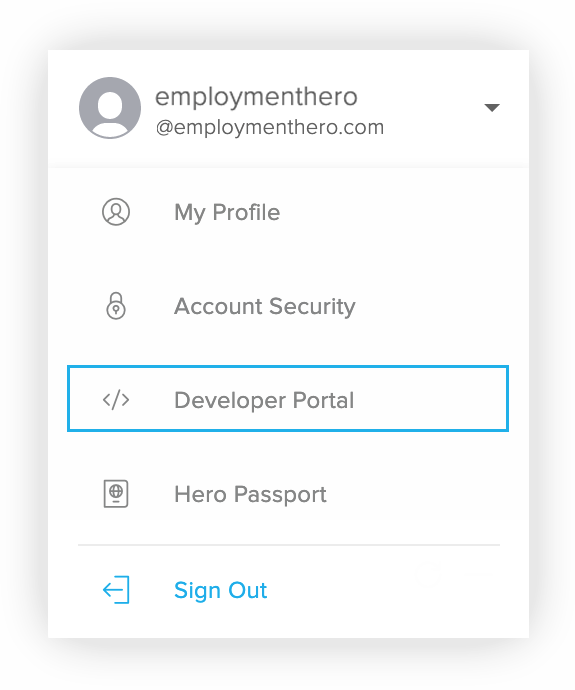
To access the developer portal, visit and sign in to your Employment Hero account. The Developer Portal link is located in the menu under your profile name in the top right corner. The developer portal includes the features:
- API (via OAuth 2.0)
- Webhooks
Obtain credentials
Visit the Developer Portal to register your application, below are the 3 fields required for creating an OAuth 2.0 application.
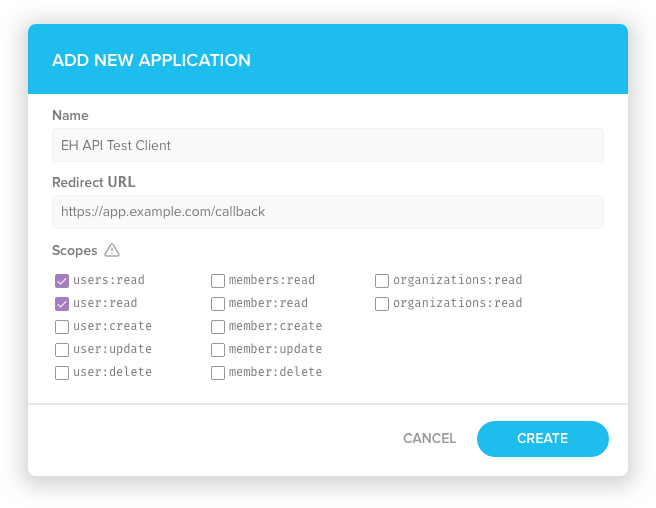
Application Properties
Field | Description |
---|---|
Name | A meaningful name for your OAuth 2.0 application (e.g. EH API Test Client) |
Scope | A list of scopes which the application can access. The scope list will be provided by Employment Hero. NOTE: For security and data sensitivity concerns, scopes are a one-time selection on application creation. |
Redirect URI(s) | One or more URIs hosted by your company which a user will be redirected to following a successful OAuth handshake. Use a comma-separated list to add multiple redirect URIs. Please note that for security purposes, we request that you provide HTTPS URIs. |
After registering a new OAuth 2.0 application, you can update your application properties except the scopes. The set of credentials includes client ID, secret and your application. Visit the application details page for the set of credentials (e.g. client ID, secret, application information) you will require in the upcoming steps. Below is the description of the generated credentials from the registration.
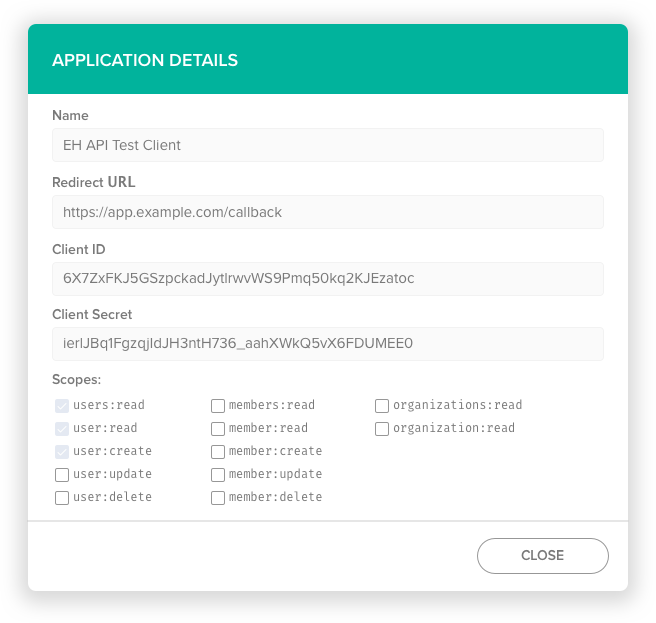
Credentials
Attribute | Description |
---|---|
Client ID | A unique string representing the registration OAuth 2.0 application. |
Client Secret | A random secret used by client to authenticate to the Employment Hero Authorisation Server. The client secret is a secret known only to the client and authorisation server. |
Obtain access token
OAuth 2.0 application credentials are used for obtaining the access token. We recommend using an admin or an owner type of account to ensure access to all relevant API endpoints.
Authorisation
Before your application can access private data over the Employment Hero API, it must obtain an access token that grants access to that API. Application scopes which are selected in the previous step controls the set of API endpoints that you are requesting for permission. A single access token is a combination of those permissions. To grant access to your application, a request must be made to our Authorisation Server.
The user used to access the application will be requested to login to Employment Hero. After logging in, the user will be asked to grant the provided permission from your OAuth 2.0 application as well as application scopes. A successful login and confirmed permissions will redirect the user back to your provided redirect url including the authorisation code. If the user does not grant the permissions, the server will return an error. The authorisation code can be used to obtain the access token.
GET
/oauth2/authorize
curl "https://oauth.employmenthero.com/oauth2/authorize? \
client_id=u6zcls9nx3b9Lq0sSLgUiTgRhMM3Miwx9KytOUrC9d0 \
&redirect_uri=https://app.example.com/callback \
&response_type=code"
RESPONSE
// example of successful logging in and granting permission
// where https://app.example.com/callback is your redirect uri
https://app.example.com/callback? \
code=7E2s5m5OHdNSPdtNMri6xNoKH62nwV_0hl9xdJ1g7fs
HTTP Request
GET https://oauth.employmenthero.com/oauth2/authorize
Params
Attribute | Description |
---|---|
client_id string |
Your client ID from OAuth 2.0 credentials |
redirect_uri string |
One of your specified redirect url(s) in your OAuth 2.0 application |
response_type string |
Use code here. |
Access Token
The access token is required to access protected resources. It represents an authorisation issued to the client and will expire after 15 minutes. To obtain it, request an access token from our Authorisation Server. The request requires client_id
, secret
, and your granted authorization_code
for security purposes. The response data includes access_token
, refresh_token
and token_type
which indicates the correct authorisation type for the access token. There are many types of authorisation when making a request, currently we only support the Bearer type.
POST
/oauth2/token
curl -X POST "https://oauth.employmenthero.com/oauth2/token? \
client_id=u6zcls9nx3b9Lq0sSLgUiTgRhMM3Miwx9KytOUrC9d0 \
&client_secret=75bAHTX7oo2ksTgnZO71BHMnmkyuaYAQzbgIeBPoecw \
&grant_type=authorization_code \
&code=7E2s5m5OHdNSPdtNMri6xNoKH62nwV_0hl9xdJ1g7fs \
&redirect_uri=https://app.example.com/callback"
RESPONSE
{
"access_token": "eyJhbGciOiJSUzI1NiJ9.joxNT...xC5vm9EwF3wDpFg",
"refresh_token": "PDhcuwQPVMU9XPVpSPekPdpbkY-mm7snmd-5nWVqjg4",
"token_type": "bearer",
"expires_in": 900,
"scope": "urn:mainapp:organisations:read urn:mainapp:employees:read"
}
HTTP Request
POST https://oauth.employmenthero.com/oauth2/token
Params
Attribute | Description |
---|---|
client_id string |
Client ID from OAuth 2.0 application credentials |
client_secret string |
Secret from OAuth 2.0 application credentials |
grant_type string |
Uses default value authorization_code |
code string |
Authorization code obtained from Authorisation Server extracted from redirect uri parameters in the previous step |
redirect_uri string |
Your redirect uri listed in the OAuth 2.0 application |
Response
Attribute | Description |
---|---|
access_token string |
The granted access token. |
refresh_token string |
The token required to refresh the access token. |
token_type string |
Type of authorisation used to access protected API. NOTE: only the Bearer type is currently supported |
expires_in number |
Lifetime of the access token (in seconds) |
scope string |
Granted scopes for this access token |
Use access token
After obtaining the access token from the Employment Hero Authorisation Server, request to the Employment Hero API must include the access token via HTTP authorisation header. All configured scopes can be seen in your OAuth 2.0 application from the Employment Hero Developer Portal page.
GET
/v1/organisations
curl "https://api.employmenthero.com/api/v1/organisations"
-H "Authorization: bearer eyJhbGciOI1NiJ9.joxNT...xC5vm9EwF3wDpFg"
Sample HTTP Request
GET https://api.employmenthero.com/api/v1/organisations
Headers
Attribute | Description |
---|---|
Authorization string |
Bearer access token obtained from Authorization Server |
Refresh the access token
Access tokens expire after 15 minutes. For continuous usage, a new access token must be requested using the refresh token.
NOTE: Refreshing the access token also returns a brand new refresh token. The previous refresh token will be invalidated.
POST
/oauth2/token
curl -X POST "https://oauth.employmenthero.com/oauth2/token? \
client_id=u6zcls9nx3b9Lq0sSLgUiTgRhMM3Miwx9KytOUrC9d0 \
&client_secret=75bAHTX7oo2ksTgnZO71BHMnmkyuaYAQzbgIeBPoecw \
&grant_type=refresh_token \
&refresh_token=lEzwW-7rz-AiLPo1ZDP0ZFEHzIwAzMbV3yfk7rsJSfs"
Response
{
"access_token": "eyJhbGciOiJSUzI1NiJ9.joxNT...xC5vm9EwF3wDpFg",
"refresh_token": "kgIaDcs5iy1jw9KN5jz84sSiGnrDr_XW5UYKwueBFDc",
"token_type": "bearer",
"expires_in": 900,
"scope": "urn:mainapp:organisations:read urn:mainapp:employees:read"
}
HTTP Request
POST https://oauth.employmenthero.com/oauth2/token
Params
Attribute | Description |
---|---|
client_id string |
Client ID from OAuth 2.0 application credentials |
client_secret string |
Secret from OAuth 2.0 application credentials |
grant_type string |
Uses default value refresh_token |
code string |
Authorisation code obtained from Authorisation Server extracted from redirect url parameters on the above step |
redirect_uri string |
Your redirect uri listed in OAuth 2.0 application |
refresh_token string |
The old refresh token obtained from access token request or last refresh token request. |
Response
Attribute | Description |
---|---|
access_token string |
The granted access token. |
refresh_token string |
The refresh token to refresh access token. |
token_type string |
Type of authorisation used to access protected API |
expires_in string |
Lifetime of the access token (in seconds) |
scope string |
Granted scopes for this access token |
Organisation
The Organisation Object
The Organisation Object
{
"data": {
"id": "bdfcb02b-fcc3-4f09-8636-c06c14345b86",
"name": "Employment Hero Pty Ltd",
"phone": "+612803222",
"country": "AU",
"logo_url": "logo.png",
"primary_address": "Test Street 79390, 11-17 York Street, SYDNEY, NSW, 2000, AU",
"end_of_week": "Saturday",
"typical_work_day": "8.0",
"payroll_admin_emails": [
"admin1@thinkei.com",
"admin2@thinkei.com",
"admin3@thinkei.com"
],
"subscription_plan": "CSA (8)",
"superfund_name": null,
"employees_count": 154,
"active_employees_count": 105,
"pending_employees_count": 4,
"time_zone": "Australia/Sydney",
"created_at": "2016-04-12T16:49:37+10:00"
}
}
Attribute | Description |
---|---|
id uuid |
Unique identifier for the object. |
name string |
The name of the organisations to retrieve |
phone string |
The phone number of your organisation |
phone string |
The phone number of your organisation |
country string |
Two-letter ISO code representing the country of your organisation |
logo_url string |
The publicly accessible URL to fetch the your organisation logo. |
primary_address string |
The organisation's address. |
end_of_week string |
The organisation's address. |
typical_work_day string |
The typical work day of your organisation (Ex: 8.0 hours). |
payroll_admin_emails array |
The list of all your payroll admin emails. |
subscription_plan string |
The organisation's subscription plan. |
superfund_name string |
The name of your organisation superfund |
employees_count number |
The number of your organisation employees |
active_employees_count number |
The number of active employees |
pending_employees_count number |
The number of pending employees |
time_zone string |
The organisation's time zone |
created_at string |
Time at which the organisation was created |
Get All Organisations
GET
/v1/organisations
curl "https://api.employmenthero.com/api/v1/organisations"
-H "Authorization: bearer AUXJ3123xyrj123fdsjkl124aAJKQ"
RESPONSE
{
"data": {
"items": [
{
"id": "bdfcb02b-fcc3-4f09-8636-c06c14345b86",
"name": "Employment Hero Pty Ltd",
"phone": "+61280302222",
"country": "AU",
"logo_url": "http://logo.png"
},
{
"id": "5a2704b0-e8a2-4765-9aec-b193e30672e4",
"name": "Thinkei",
"phone": "+611234567890",
"country": "AU",
"logo_url": "http://logo.png"
}
],
"item_per_page": 20,
"page_index": 1,
"total_pages": 1,
"total_items": 2
}
}
Returns an array of all organisations. Every organisation must be managed by you or the organisation which you work for.
HTTP Request
GET https://api.employmenthero.com/api/v1/organisations
Return
A hash with a data property that contains an item array of up to limit organisations. Each entry in the array is a separate organisation object. If there are no more organisations, the resulting array will be empty.
Get An Organisation
GET
/v1/organisations/:id
curl "https://api.employmenthero.com/api/v1/organisations/bdfcb02b-fcc3-4f09-8636-c06c14345b86"
-H "Authorization: bearer AUXJ3123xyrj123fdsjkl124aAJKQ"
RESPONSE
{
"data": {
"id": "bdfcb02b-fcc3-4f09-8636-c06c14345b86",
"name": "Employment Hero Pty Ltd",
"phone": "+612803222",
"country": "AU",
"logo_url": "logo.png",
"primary_address": "Test Street 79390, 11-17 York Street, SYDNEY, NSW, 2000, AU",
"end_of_week": "Saturday",
"typical_work_day": "8.0",
"payroll_admin_emails": [
"admin1@thinkei.com",
"admin2@thinkei.com",
"admin3@thinkei.com"
],
"subscription_plan": "CSA (8)",
"superfund_name": null,
"employees_count": 154,
"active_employees_count": 105,
"pending_employees_count": 4,
"time_zone": "Australia/Sydney",
"created_at": "2016-04-12T16:49:37+10:00"
}
}
This endpoint retrieves information for a specific organisation. The same data is returned as when listing the organisation, but with some additional details.
HTTP Request
GET https://api.employmenthero.com/api/v1/organisations/:organisation_id
Parameter | Description |
---|---|
organisation_id uuid |
The ID of the organisations to retrieve |
Employee
The Employee Object
The Employee Object
{
"id": "0139ebb7-6f3e-4bc0-954e-9e50614fccd1",
"account_email": "daniel@thinkei.com",
"title": "Mr",
"role": "owner",
"first_name": "Daniel",
"last_name": "Nguyen",
"middle_name": "",
"address": "Test Street 184752, SYDNEY, NSW, 2000, AU",
"avatar_url": "http://avatar.jpg",
"known_as": "",
"job_title": "Grad Developer",
"gender": "Female",
"country": "AU",
"nationality": "",
"date_of_birth": "1995-02-13T00:00:00+00:00",
"marital_status": "",
"personal_email": "daniel.nguyen@thinkei.com",
"personal_mobile_number": "01285659993",
"home_phone": "",
"employing_entity": "US",
"code": "",
"location": null,
"company_email": "daniel.nguyen@thinkei.com",
"company_mobile": "",
"company_landline": "",
"start_date": "2017-03-01T00:00:00+00:00",
"termination_date": null,
"primary_cost_centre": {
"id": "95df8139-479f-432e-b8f9-922352d2fe4a",
"name": "Employment Hero"
},
"secondary_cost_centres": [],
"primary_manager": {
"id": "4a728243-8930-4a93-9bd8-a6843e7b59ec",
"name": "Jessica"
},
"secondary_manager": null,
"external_id": "external_id_123"
}
Attribute | Description |
---|---|
id uuid |
Unique identifier for the object. |
account_name string |
The account name of employee |
title string |
The title of employee |
role string |
The role of employee |
first_name string |
The first name of employee |
last_name string |
The last name of employee |
middle_name string |
The middle name of employee |
address string |
The address of employee |
avatar_url string |
The avatar url of employee |
known_as string |
The another identity of employee |
job_title string |
The job title of employee |
gender string |
Employee's gender |
country string |
Employee's country |
date_of_birth string |
The date of birth of employee |
marital_status string |
The martial status of employee |
personal_email string |
The personal email of employee |
personal_mobile_number string |
The personal mobile number of employee |
home_phone string |
The home phone of employee |
employing_entity string |
The employing entity of employee |
location string |
The location of employee |
company_email string |
The company email of employee |
company_mobile string |
The company mobile of employee |
start_date string |
The start_date of employee |
termination_date string |
The termination day of employee |
primary_cost_centre object |
The primary cost centre of employee |
secondary_cost_centre array |
The secondary cost centre of employee |
primary_manager object |
The primary manager of employee |
secondary_manager object |
The secondary manager of employee |
external_id string |
The external_id of employee |
Get Employees
GET
/v1/organisations/:id/employees
curl "https://api.employmenthero.com/api/v1/organisations/:id/employees"
-H "Authorization: bearer AUXJ3123xyrj123fdsjkl124aAJKQ"
RESPONSE
{
"data": {
"items": [
{
"id": "0139ebb7-6f3e-4bc0-954e-9e50614fccd1",
"account_email": "daniel@thinkei.com",
"title": "Ms",
"role": "owner",
"first_name": "Daniel",
"last_name": "Tran",
"middle_name": "",
"address": "Test Street 184752, SYDNEY, NSW, 2000, AU",
"avatar_url": "http://avatar.png",
"known_as": "",
"job_title": "Grad Developer",
"gender": "Female",
"country": "AU",
"nationality": "",
"date_of_birth": "1995-02-13T00:00:00+00:00",
"marital_status": "",
"personal_email": "abc@thinkei.com",
"personal_mobile_number": "01285659993",
"home_phone": "",
"employing_entity": "US",
"code": "",
"location": null,
"company_email": "abc@thinkei.com",
"company_mobile": "",
"company_landline": "",
"start_date": "2017-03-01T00:00:00+00:00",
"termination_date": null,
"primary_cost_centre": {
"id": "95df8139-479f-432e-b8f9-922352d2fe4a",
"name": "Employment Hero"
},
"secondary_cost_centres": [],
"primary_manager": {
"id": "4a728243-8930-4a93-9bd8-a6843e7b59ec",
"name": "Jessica"
},
"secondary_manager": null,
"external_id": "123External"
}
]
}
}
Returns an array of all employees. Every employees must be managed by your managed organisation.
HTTP Request
GET https://api.employmenthero.com/api/v1/organisations/:organisation_id/employees
Return
A hash with a data property that contains an array of up to the limit of employees. Each entry in the array is a separate employee object. If there are no more employees belonging to your organisation, the resulting array will be empty.
Query Parameters
Parameter | Description |
---|---|
organisation_id uuid |
The ID of the organisation to get employees |
Get An Employee
GET
/v1/organisations/:id
curl "https://api.employmenthero.com/api/v1/organisations/:organisation_id/employees/:id"
-H "Authorization: bearer AUXJ3123xyrj123fdsjkl124aAJKQ"
RESPONSE
{
"data": {
"id": "0139ebb7-6f3e-4bc0-954e-9e50614fccd1",
"account_email": "daniel@thinkei.com",
"title": "Mr",
"role": "owner",
"first_name": "Daniel",
"last_name": "Nguyen",
"middle_name": "",
"address": "Test Street 184752, SYDNEY, NSW, 2000, AU",
"avatar_url": "http://avatar.jpg",
"known_as": "",
"job_title": "Grad Developer",
"gender": "Female",
"country": "AU",
"nationality": "",
"date_of_birth": "1995-02-13T00:00:00+00:00",
"marital_status": "",
"personal_email": "daniel.nguyen@thinkei.com",
"personal_mobile_number": "01285659993",
"home_phone": "",
"employing_entity": "US",
"code": "",
"location": null,
"company_email": "daniel.nguyen@thinkei.com",
"company_mobile": "",
"company_landline": "",
"start_date": "2017-03-01T00:00:00+00:00",
"termination_date": null,
"primary_cost_centre": {
"id": "95df8139-479f-432e-b8f9-922352d2fe4a",
"name": "Employment Hero"
},
"secondary_cost_centres": [],
"primary_manager": {
"id": "4a728243-8930-4a93-9bd8-a6843e7b59ec",
"name": "Jessica"
},
"secondary_manager": null,
"external_id": "external_id_123"
}
}
This endpoint retrieves a specific employee.
HTTP Request
GET https://api.employmenthero.com/api/v1/organisations/:organisation_id/employees/:id
Parameter | Description |
---|---|
id uuid |
The ID of the employee to retrieve |
organisation_id uuid |
The ID of the organisation to retrieve |
Leave Request
The Leave Request Object
The Leave Request Object
{
"id": "724ec1f7-ebe9-4af6-84bf-7f071167007a",
"start_date": "2017-05-29T00:00:00+00:00",
"end_date": "2017-05-29T00:00:00+00:00",
"total_hours": 8.0,
"comment": "The last working day",
"status": "Approved",
"leave_balance_amount": 0.0,
"leave_category_name": "Annual Leave",
"reason": null,
"employee_id": "0139ebb7-6f3e-4bc0-954e-9e50614fccd1"
}
Attribute | Description |
---|---|
id uuid |
Unique identifier for the object. |
start_date string |
The start date of your employee leave request. |
end_date string |
The end date of your employee leave request. |
total_hours number |
The total hours of leave request. |
comment string |
The comment of the leave request if an owner or admin has entered a notation |
status string |
The status of leave request. There are only 3 status "Approved", "Declined", "Pending" |
leave_balance_amount number |
The leave balance amount of leave request. |
leave_category_name string |
The category name of leave request object. I.E "Annual Leave" |
reason string |
The reason of employee's leave request. |
employee_id uuid |
The ID of employee who requested to leave. |
Get Leave Requests
GET
/v1/organisations/:organisation_id/leave_requests
curl
"https://api.employmenthero.com/api/v1/organisations/:organisation_id/leave_requests"
-H "Authorization: bearer AUXJ3123xyrj123fdsjkl124aAJKQ"
RESPONSE
{
"data": {
"items": [
{
"id": "724ec1f7-ebe9-4af6-84bf-7f071167007a",
"start_date": "2017-05-29T00:00:00+00:00",
"end_date": "2017-05-29T00:00:00+00:00",
"total_hours": 8.0,
"comment": "The last working day",
"status": "Approved",
"leave_balance_amount": 0.0,
"leave_category_name": "Annual Leave",
"reason": "Vacation",
"employee_id": "0139ebb7-6f3e-4bc0-954e-9e50614fccd1"
}
]
}
}
This endpoint retrieves a list of all leave requests.
HTTP Request
GET https://api.employmenthero.com/api/v1/organisations/:organisation_id/leave_requests
Parameter | Description |
---|---|
organisation_id uuid |
The ID of the organisation to retrieve |
Return
A hash with a data property that contains an array of up to the limit of leave requests. Each entry in the array is a separate leave request object. If there are no more leave requests, the resulting array will be empty.
Get A Leave Request
GET
/v1/organisations/:organisation_id/leave_requests/:id
curl "https://api.employmenthero.com/api/v1/organisations/:organisation_id/leave_requests/:id"
-H "Authorization: bearer AUXJ3123xyrj123fdsjkl124aAJKQ"
RESPONSE
{
"data": {
"id": "724ec1f7-ebe9-4af6-84bf-7f071167007a",
"start_date": "2017-05-29T00:00:00+00:00",
"end_date": "2017-05-29T00:00:00+00:00",
"total_hours": 8.0,
"comment": "The last working day",
"status": "Approved",
"leave_balance_amount": 0.0,
"leave_category_name": "Annual Leave",
"reason": "Vacation",
"employee_id": "0139ebb7-6f3e-4bc0-954e-9e50614fccd1"
}
}
This endpoint retrieves a specific leave request.
HTTP Request
GET https://api.employmenthero.com/api/v1/organisations/:organisation_id/leave_requests/:id
Parameter | Description |
---|---|
id uuid |
The ID of the leave request to retrieve |
organisation_id uuid |
The ID of the organisation to retrieve |
Timesheet Entry
The Timesheet Object
The Timesheet Object
{
"id": "16a62c0d-bb91-45e4-ad47-a325117c87eb",
"date": "2018-12-17T00:00:00+00:00",
"start_time": "2018-12-17T01:00:00+11:00",
"end_time": "2018-12-17T07:00:00+11:00",
"status": "pending",
"units": 6.0,
"reason": null,
"comment": "Working hard",
"time": 21600
}
Attribute | Description |
---|---|
id uuid |
Unique identifier for the object. |
date string |
The date of your employee timesheet object. |
start_time string |
The start time of timesheet. |
end_time string |
The end time of timesheet. |
status string |
The status of timesheet. There are only 3 supported statuses pending , approved , rejected |
units number |
The number of hours for this timesheet record |
reason string |
The reason of timesheet. |
comment string |
The comment of timesheet |
time number |
The time of timesheet (miliseconds), if start_time is empty or end_time is empty then this field will have the value |
Get Timesheet Entries
GET
/v1/organisations/:organisation_id/employees/:employee_id/timesheet_entries
curl
"https://api.employmenthero.com/api/v1/organisations/:organisation_id/employees/:employee_id/timesheet_entries"
-H "Authorization: bearer AUXJ3123xyrj123fdsjkl124aAJKQ"
RESPONSE
{
"data": {
"items": [
{
"id": "16a62c0d-bb91-45e4-ad47-a325117c87eb",
"employee_id": "001c20e3-c752-4a46-81a1-a5e476e42d06",
"date": "2018-12-17T00:00:00+00:00",
"start_time": "2018-12-17T01:00:00+11:00",
"end_time": "2018-12-17T07:00:00+11:00",
"status": "pending",
"units": 6.0,
"reason": null,
"comment": "",
"time": 21600,
"cost_centre": {
"id": "4a4f1ad1-bf2d-4d6c-b620-6557911f85cc",
"name": "R&D"
}
},
{
"id": "9719cfda-3e54-4e31-b105-d306e0371546",
"employee_id": "001c20e3-c752-4a46-81a1-a5e476e42d06",
"date": "2019-03-30T00:00:00+00:00",
"start_time": null,
"end_time": null,
"status": "approved",
"units": 3.0,
"reason": null,
"comment": "",
"time": 10800,
"cost_centre": {
"id": "4a4f1ad1-bf2d-4d6c-b620-6557911f85cc",
"name": "R&D"
}
}
],
"item_per_page": 20,
"page_index": 1,
"total_pages": 1,
"total_items": 2
}
}
This endpoint retrieves a list of all timesheet entries for a specific employee.
HTTP Request
GET https://api.employmenthero.com/api/v1/organisations/:organisation_id/employees/:employee_id/timesheet_entries?start_date=&end_date=
Parameter | Description |
---|---|
employee_id uuid |
The ID of the employee to retrieve, we also support the wildcard collection id "-" for searching for all timesheet across all employees |
organisation_id uuid |
The ID of the organisation to retrieve |
start_date string |
The start date of time range for date field (dd/mm/yyyy) |
end_date string |
The end date of time range for date field (dd/mm/yyyy) |
Return
A hash with a data property that contains an array of up to the limit of timesheets. Each entry in the array is a separate timesheet object. If there are no more timesheets, the resulting array will be empty.
Employment History
The Employment History Object
The Employment History Object
{
"id": "bf9c12e1-b40b-4fa9-abcb-d824e5cb3654",
"title": "Grad Developer",
"start_date": "2017-03-01T00:00:00+00:00",
"end_date": null,
"employment_type": "Full-time"
}
Attribute | Description |
---|---|
id uuid |
Unique identifier for the object. |
title string |
The title of employee |
start_date string |
The start date of employment history |
end_date string |
The end date of employment history |
employment_type string |
The employment type of employee, there are only 3 types Full-time , Part-time , Casual |
Get Employment History
GET
/v1/organisations/:organisation_id/employees/:employee_id/employment_histories
curl
"https://api.employmenthero.com/api/v1/organisations/:organisation_id/employees/:employee_id/employment_histories"
-H "Authorization: bearer AUXJ3123xyrj123fdsjkl124aAJKQ"
RESPONSE
{
"data": {
"items": [
{
"id": "bf9c12e1-b40b-4fa9-abcb-d824e5cb3654",
"title": "Grad Developer",
"start_date": "2017-03-01T00:00:00+00:00",
"end_date": null,
"employment_type": "Full-time"
}
],
"item_per_page": 20,
"page_index": 1,
"total_pages": 1,
"total_items": 1
}
}
This endpoint retrieves a list of the complete employment history for a specific employee.
HTTP Request
GET
https://api.employmenthero.com/api/v1/organisations/:organisation_id/employees/:employee_id/employment_histories
Parameter | Description |
---|---|
employee_id uuid |
The ID of the employee to retrieve |
organisation_id uuid |
The ID of the organisation to retrieve |
Return
A hash with a data property that contains an array of up to the limit of employment history records. Each entry in the array is a separate employment history object. If there is no more employment history, the resulting array will be empty.
Emergency Contact
The Emergency Contact Object
The Emergency Contact Object
{
"id": 110762,
"contact_name": "Daniel Levi",
"daytime_contact_number": "02222222222",
"after_hours_no": "02222222222",
"after_mobile_no": "02222222222",
"relationship": "wife",
"contact_type": "primary"
}
Attribute | Description |
---|---|
id uuid |
Unique identifier for the object. |
contact_name string |
The name of contactor |
daytime_contact_number string |
The phone number of contactor in daytime |
after_hours_no string |
The phone number of contactor after working hours |
after_mobile_no string |
the mobile number of contactor after working hours |
relationship string |
The relationship between contactor and the employee |
contact_type string |
The type of contactor |
Get Emergency Contact
GET
/v1/organisations/:organisation_id/employees/:employee_id/emergency_contacts
curl
"https://api.employmenthero.com/api/v1/organisations/:organisation_id/employees/:employee_id/emergency_contacts"
-H "Authorization: bearer AUXJ3123xyrj123fdsjkl124aAJKQ"
RESPONSE
{
"data": {
"items": [
{
"id": 110762,
"contact_name": "Daniel Levi",
"daytime_contact_number": "02222222222",
"after_hours_no": "02222222222",
"after_mobile_no": "02222222222",
"relationship": "wife",
"contact_type": "primary"
}
],
"item_per_page": 20,
"page_index": 1,
"total_pages": 1,
"total_items": 1
}
}
This endpoint retrieves a list of all emergency contacts for a specific employee.
HTTP Request
GET
https://api.employmenthero.com/api/v1/organisations/:organisation_id/employees/:employee_id/emergency_contacts
Parameter | Description |
---|---|
employee_id uuid |
The ID of the employee to retrieve |
organisation_id uuid |
The ID of the organisation to retrieve |
Return
A hash with a data property that contains an array of up to the limit of emergency contacts. Each entry in the array is a separate emergency contact object. If there are no more emergency contacts, the resulting array will be empty.
Team
The Team Object
The Team Object
{
"id": "143ed07a-e7d1-4c19-ade6-b0ffe9123d19",
"name": "Customer Success",
"status": "active"
}
Attribute | Description |
---|---|
id uuid |
Unique identifier for the object. |
name string |
The name of the team to retrieve |
status string |
The status of your team, there are only 2 supported statuses active , disabled |
Get All Team
GET
/v1/organisations/:organisation_id/teams
curl "https://api.employmenthero.com/api/v1/organisations/:organisation_id/teams"
-H "Authorization: bearer AUXJ3123xyrj123fdsjkl124aAJKQ"
RESPONSE
{
"data": {
"items": [
{
"id": "143ed07a-e7d1-4c19-ade6-b0ffe9123d19",
"name": "Customer Success",
"status": "active"
},
{
"id": "36bd330e-3a6b-4539-8c86-a414fc3b485e",
"name": "Engineering",
"status": "active"
},
{
"id": "7a040b66-9bd7-47d4-b6e4-c68ed0931876",
"name": "Finance",
"status": "active"
}
],
"item_per_page": 20,
"page_index": 1,
"total_pages": 1,
"total_items": 3
}
}
Returns an array of all teams. Every teams must be managed by one of your managed organisation.
HTTP Request
GET https://api.employmenthero.com/api/v1/organisations/:organisation_id/teams
Parameter | Description |
---|---|
organisation_id uuid |
The ID of organisation which the all teams belong to |
Return
A hash with a data property that contains an array of up to the limit of teams. Each entry in the array is a separate team object. If there are no more teams belonging to your organisation, the resulting array will be empty.
Get All Employees of a Team
GET
/v1/organisations/:organisation_id/teams/:team_id/employees
curl "https://api.employmenthero.com/api/v1/organisations/:organisation_id/teams/:team_id/employees"
-H "Authorization: bearer AUXJ3123xyrj123fdsjkl124aAJKQ"
RESPONSE
{
"data": {
"items": [
{
"id": "f69ff409-9d61-40c4-ac89-7f840238b0c5",
"account_email": "daniel@employmenthero.com",
"title": "Mr",
"role": "owner",
"first_name": "Daniel",
"last_name": "Janes",
"middle_name": "",
"address": "Test Street 184752, SYDNEY, NSW, 2000, AU",
"avatar_url": "http://avatar.png",
"known_as": "Daniel",
"job_title": "Sales Manager",
"gender": "Male",
"country": "AU",
"nationality": "",
"date_of_birth": "1992-09-14T00:00:00+00:00",
"marital_status": "",
"personal_email": "daniel@employmenthero.com",
"personal_mobile_number": "",
"home_phone": "",
"employing_entity": "Employment Hero",
"code": "9999",
"location": null,
"company_email": "eh@employmenthero.com",
"company_mobile": "",
"company_landline": "",
"start_date": "2020-02-01T00:00:00+00:00",
"termination_date": null,
"primary_cost_centre": {
"id": "95df8139-479f-432e-b8f9-922352d2fe4a",
"name": "Employment Hero"
},
"secondary_cost_centres": [],
"primary_manager": {
"id": "4a728243-8930-4a93-9bd8-a6843e7b59ec",
"name": "Jessica"
},
"secondary_manager": null,
"external_id": "123External"
}
],
"item_per_page": 20,
"page_index": 1,
"total_pages": 1,
"total_items": 3
}
}
Returns an array of employees associated to a specific team. Employees must be managed by your managed organisation.
The Employee Object is defined here
HTTP Request
GET https://api.employmenthero.com/api/v1/organisations/:organisation_id/teams/:team_id/employees
Query Parameters
Parameter | Description |
---|---|
organisation_id uuid |
The ID of the organisation to get employees |
team_id uuid |
The ID of the team to get employees |
Return
A hash with a data property that contains an item array of up to limit employees. Each entry in the array is a separate employee object. If there are no more employees, the resulting array will be empty.
Bank Account
The Bank Account Object
The Bank Account Object
{
"id": "6ddbfb24-8153-42a9-9248-c41c9b6c755e",
"account_name": "Daniel Nguyen",
"account_number": "111111111",
"bsb": "111111",
"amount": 100.0,
"primary_account": true
}
Attribute | Description |
---|---|
id uuid |
Unique identifier for the object. |
account_name string |
The account name of a bank account. |
account_number string |
The account number of a bank account. |
bsb string |
The bsb number of a bank account. |
amount number |
The amount of a bank account. |
primary_account boolean |
The boolean flag to determine whether this bank account is primary or not. |
Get Bank Accounts
GET
/v1/organisations/:organisation_id/employees/:employee_id/bank_accounts
curl
"https://api.employmenthero.com/api/v1/organisations/:organisation_id/employees/:employee_id/bank_accounts"
-H "Authorization: bearer AUXJ3123xyrj123fdsjkl124aAJKQ"
RESPONSE
{
"data": {
"items": [
{
"id": "6ddbfb24-8153-42a9-9248-c41c9b6c755e",
"account_name": "Daniel Nguyen",
"account_number": "111111111",
"bsb": "111111",
"amount": 100.0,
"primary_account": true,
"external_id": null
}
],
"item_per_page": 20,
"page_index": 1,
"total_pages": 1,
"total_items": 1
}
}
This endpoint retrieves a list of all bank accounts for a specific employee.
HTTP Request
GET https://api.employmenthero.com/api/v1/organisations/:organisation_id/employees/:employee_id/bank_accounts
Parameter | Description |
---|---|
employee_id uuid |
The ID of the employee to retrieve |
organisation_id uuid |
The ID of the organisation to retrieve |
Return
A hash with a data property that contains an array of up to the limit of bank account records. Each entry in the array is a separate bank account object. If there is no more bank accounts, the resulting array will be empty.
Policy
The Policy Object
The Policy Object
{
"id": "1d25a390-7b0e-0135-0209-061f87a4b1b6",
"name": "Wonderful Company - Full-Disk Encryption Policy",
"induction": true,
"created_at": "2017-09-14T10:03:51+10:00"
}
Attribute | Description |
---|---|
id uuid |
The policy id |
name string |
The name of policy |
induction boolean |
Determine policy is mandatory or not |
created_at string |
Time at which the policy was created |
Get Policies
GET
/v1/organisations/:organisation_id/polices
curl
"https://api.employmenthero.com/api/v1/organisations/:organisation_id/policies"
-H "Authorization: bearer AUXJ3123xyrj123fdsjkl124aAJKQ"
RESPONSE
{
"data": {
"items": [
{
"id": "1d25a390-7b0e-0135-0209-061f87a4b1b6",
"name": "Wonderful Company - Full-Disk Encryption Policy",
"induction": true,
"created_at": "2017-09-14T10:03:51+10:00"
},
{
"id": "91216a90-f3cb-0133-ec72-4a8f8c38fb42",
"name": "Wonderful Company - Working From Home Agreement",
"induction": false,
"created_at": "2016-05-04T12:12:25+10:00"
}
],
"item_per_page": 20,
"page_index": 1,
"total_pages": 2,
"total_items": 2
}
}
This endpoint retrieves a list of all polices for a specific organisation.
HTTP Request
GET https://api.employmenthero.com/api/v1/organisations/:organisation_id/policies
URL Parameters
Parameter | Description |
---|---|
organisation_id uuid |
The ID of the organisation to retrieve |
Return
A hash with a data property that contains an array of up to the limit of policy records. Each entry in the array is a separate policy object. If there is no more policies, the resulting array will be empty.
Certification
The Certification Object
The Certification Object
{
"id": "3128a2bb-2b34-4437-9a00-92af3dab5b59",
"name": "Employment Hero System Training",
"type": "check"
}
Attribute | Description |
---|---|
id uuid |
The certification id |
name string |
The name of certification |
type string |
The type of certification, there are only 4 supported types check , licence , qualification , training |
Get All Certifications
GET
/v1/organisations/:organisation_id/certifications
curl "https://api.employmenthero.com/api/v1/organisations/:organisation_id/certifications"
-H "Authorization: bearer AUXJ3123xyrj123fdsjkl124aAJKQ"
RESPONSE
{
"data": {
"items": [
{
"id": "6538a2bb-2b34-4437-9a00-92af3dab5b59",
"name": "Full-disk encryption",
"status": "check"
},
{
"id": "3128a2bb-2b34-4437-9a00-92af3dab5b59",
"name": "System Training",
"status": "training"
},
{
"id": "s562a2bb-2b34-4437-9a00-92af3dab5b59",
"name": "Staff training",
"status": "training"
}
],
"item_per_page": 20,
"page_index": 1,
"total_pages": 1,
"total_items": 3
}
}
This endpoint retrieves a list of all certification of a specific organisation.
HTTP Request
GET https://api.employmenthero.com/api/v1/organisations/:organisation_id/certifications
Parameter | Description |
---|---|
organisation_id uuid |
The ID of the organisation to retrieve |
Return
A hash with a data property that contains an array of up to the limit of certifications. Each entry in the array is a separate certification object. If there are no more certifications, the resulting array will be empty.
Get A Certification
GET
/v1/organisations/:organisation_id/certifications/:id
curl "https://api.employmenthero.com/api/v1/organisations/:organisation_id/certifications/:id"
-H "Authorization: bearer AUXJ3123xyrj123fdsjkl124aAJKQ"
RESPONSE
{
"data": {
"id": "3128a2bb-2b34-4437-9a00-92af3dab5b59",
"name": "Full-disk encryption",
"type": "check"
}
}
This endpoint retrieves a specific certification.
HTTP Request
GET https://api.employmenthero.com/api/v1/organisations/:organisation_id/certifications/:id
Query Parameters
Attribute | Description |
---|---|
id uuid |
The certification id |
organisation_id uuid |
The ID of the organisation to retrieve |
Employee Certification Detail
The Employee Certification Object
The Employee Certification Object
{
"id": "3128a2bb-2b34-4437-9a00-92af3dab5b59",
"name": "Full-disk encryption",
"certification_id": "6538a2bb-2b34-4437-9a00-92af3dab5b33",
"type": "Check",
"expiry_date": "2021-01-04T00:00:00+00:00",
"completion_date": "2020-01-04T00:00:00+00:00",
"driver_problem": false,
"driver_details": "",
"status": "Outstanding",
"reason": ""
}
Attribute | Description |
---|---|
id uuid |
The employee certification id |
name string |
The certification name of employee certification |
certification_id string |
The certification id of employee certification |
type string |
The certification type of employee certification |
expiry_date string |
The expiry date of employee certification |
completion_date string |
The completion date of employee certification |
driver_problem boolean |
The certification driver problem of employee certification |
driver_details string |
The certification driver details of employee certification |
status string |
The status of certification, there are only 4 supported statuses Outstanding , In review , Approved , Declined , Expired |
reason string |
The certification reason of employee certification |
Get All Employee Certification Details
GET
/v1/organisations/:organisation_id/employees/:employee_id/certifications
curl "https://api.employmenthero.com/api/v1/organisations/:organisation_id/employees/:employee_id/certifications"
-H "Authorization: bearer AUXJ3123xyrj123fdsjkl124aAJKQ"
RESPONSE
{
"data": {
"items": [
{
"id": "3128a2bb-2b34-4437-9a00-92af3dab5b59",
"name": "Full-disk encryption",
"certification_id": "6538a2bb-2b34-4437-9a00-92af3dab5b33",
"type": "Check",
"expiry_date": "2021-01-04T00:00:00+00:00",
"completion_date": "2020-01-04T00:00:00+00:00",
"driver_problem": false,
"driver_details": "",
"status": "Outstanding",
"reason": ""
},
{
"id": "9c74cd5c-0bbd-4b81-afa4-ef21786f7767",
"name": "OKR staff training",
"certification_id": "6538a2bb-2b34-4437-9a00-92af3dab5byy",
"type": "Training",
"expiry_date": "2020-02-01T00:00:00+00:00",
"completion_date": "2019-02-01T00:00:00+00:00",
"driver_problem": false,
"driver_details": "",
"status": "In review",
"reason": ""
},
{
"id": "0384b371-394f-458c-95be-8804d3cc02cf",
"name": "Employment Hero System Training",
"certification_id": "6538a2bb-2b34-4437-9a00-2af3dab59873",
"type": "Training",
"expiry_date": "2021-05-01T00:00:00+00:00",
"completion_date": "2020-05-01T00:00:00+00:00",
"driver_problem": true,
"driver_details": "",
"status": "Declined",
"reason": ""
}
],
"item_per_page": 20,
"page_index": 1,
"total_pages": 1,
"total_items": 3
}
}
This endpoint retrieves a list of all certifications assigned to a specific employee.
HTTP Request
GET https://api.employmenthero.com/api/v1/organisations/:organisation_id/employees/:employee_id/certifications
Parameter | Description |
---|---|
organisation_id uuid |
The ID of the organisation to retrieve |
employee_id uuid |
The ID of the employee to retrieve |
Return
A hash with a data property that contains an item array of up to limit employee certifications. Each entry in the array is a separate employee certification object. If there are no more employee certification, the resulting array will be empty.
Payslip
The Payslip Object
The Payslip Object
{
"id": "e27387ba-2105-4d12-be95-a7e74823f70f",
"first_name": "Daniel",
"last_name": "Nguyen",
"total_deduction": 1280.5,
"net_pay": 2520.15,
"super": 301.15,
"wages": 3346.15,
"reimbursements": 1280.5,
"tax": 826.0,
"name": "Alex Kopczynski",
"address_line1": "4 Sava",
"address_line2": null,
"suburb": "123 sydney",
"postcode": "0880",
"address_state": "NT",
"note": null,
"post_tax_deduction": 0.0,
"pre_tax_deduction": 0.0,
"business_name": "One Disease Limited",
"business_address": "Australia",
"business_abn": "57162909284",
"base_rate": 87000.0,
"hourly_rate": 0.0,
"pay_period_starting": "2012-06-27T00:00:00+00:00",
"pay_period_ending": "2012-07-10T00:00:00+00:00",
"base_rate_unit": "Annually",
"employment_type": "Annually",
"payroll_type": null,
"payment_date": "2012-07-11T00:00:00+10:00"
}
Attribute | Description |
---|---|
id uuid |
Unique identifier for the object. |
first_name string |
The employee first name. |
last_name string |
The employee last name. |
total_deduction number |
The total amount of deductions for the period. |
net_pay number |
The net pay for the period. |
super number |
The super contribution for the period. |
wages number |
The total wage for the period. |
reimbursements number |
The total amount of expense reimbursements for the pay period. |
tax number |
The total tax for the period. |
name string |
The full name of the employee. |
address_line1 string |
The employee address 1. |
address_line2 string |
The employee address 2. |
suburb string |
The employee suburb. |
postcode string |
The employee postcode. |
address_state string |
The employee address state. |
note string |
The payslip note. |
post_tax_deduction number |
The total amount of post tax deductions for the period. |
pre_tax_deduction number |
The total amount of pre tax deductions for the period. |
business_name string |
The name of the business. |
business_address string |
The address of the business. |
business_abn string |
The business number. |
base_rate number |
The employee's base rate. |
hourly_rate number |
The employee's hourly rate. |
pay_period_starting string |
The start date of the pay period. |
pay_period_ending string |
The end date of the pay period. |
base_rate_unit string |
The unit of pay for the base rate. |
employment_type string |
The employee's employment type. |
payroll_type string |
The type of payroll. |
payment_date string |
The payment date. |
Get Payslips
GET
/v1/organisations/:organisation_id/employees/:employee_id/payslips
curl
"https://api.employmenthero.com/api/v1/organisations/:organisation_id/employees/:employee_id/payslips"
-H "Authorization: bearer AUXJ3123xyrj123fdsjkl124aAJKQ"
RESPONSE
{
"data": {
"items": [
{
"id": "e27387ba-2105-4d12-be95-a7e74823f70f",
"first_name": "Daniel",
"last_name": "Nguyen",
"total_deduction": 1280.5,
"net_pay": 2520.15,
"super": 301.15,
"wages": 3346.15,
"reimbursements": 1280.5,
"tax": 826.0,
"name": "Alex Kopczynski",
"address_line1": "4 Sava",
"address_line2": null,
"suburb": "123 sydney",
"postcode": "0880",
"address_state": "NT",
"note": null,
"post_tax_deduction": 0.0,
"pre_tax_deduction": 0.0,
"business_name": "One Disease Limited",
"business_address": "Australia",
"business_abn": "57162909284",
"base_rate": 87000.0,
"hourly_rate": 0.0,
"pay_period_starting": "2012-06-27T00:00:00+00:00",
"pay_period_ending": "2012-07-10T00:00:00+00:00",
"base_rate_unit": "Annually",
"employment_type": "Annually",
"payroll_type": null,
"payment_date": "2012-07-11T00:00:00+10:00"
}
],
"item_per_page": 20,
"page_index": 1,
"total_pages": 1,
"total_items": 1
}
}
This endpoint retrieves a list of all payslips for a specific employee.
HTTP Request
GET https://api.employmenthero.com/api/v1/organisations/:organisation_id/employees/:employee_id/payslips
URL Parameters
Parameter | Description |
---|---|
organisation_id uuid |
The ID of the organisation to retrieve |
employee_id uuid |
The ID of the employee to retrieve |
Return
A hash with a data property that contains an array of up to the limit of payslip records. Each entry in the array is a separate payslip object. If there is no more payslips, the resulting array will be empty.
Pay Detail
The Pay Detail Object
The Pay Detail Object
{
"id": "7d9e663f-a493-4523-aea4-4f299efee8b8",
"effective_from": "2020-04-21",
"classification": "Day Working - Full Time",
"industrial_instrument": "Wholesale Award 2010",
"pay_rate_template": "Permanent Storeworker",
"anniversary_date": "2020-03-25T00:00:00+00:00",
"salary": 50,
"salary_type": "Hour",
"pay_unit": "Hourly",
"pay_category": "Permanent Ordinary Hours",
"leave_allowance_template": "Permanent",
"change_reason": "Some reasons",
"comments": "Some comments"
}
Attribute | Description |
---|---|
id uuid |
Unique identifier for the object. |
effective_from string |
The effect date of the pay detail. |
classification string |
The name of the classification. |
industrial_instrument string |
The name of the industrial instrument. |
pay_rate_template string |
The name of the pay rate template. |
anniversary_date string |
The anniversary date of the pay detail. |
salary number |
The salary amount. |
salary_type string |
The type of salary. |
pay_unit string |
The unit of pay rate. |
pay_category string |
The name of the pay category. |
leave_allowance_template string |
The name of the leave allowance template. |
change_reason string |
The reason of changing pay detail. |
comments string |
The comments. |
Get Pay Details
GET
/v1/organisations/:organisation_id/employees/:employee_id/pay_details
curl
"https://api.employmenthero.com/api/v1/organisations/:organisation_id/employees/:employee_id/pay_details"
-H "Authorization: bearer AUXJ3123xyrj123fdsjkl124aAJKQ"
RESPONSE
{
"data": {
"items": [
{
"id": "7d9e663f-a493-4523-aea4-4f299efee8b8",
"effective_from": "2020-04-21",
"classification": "Day Working - Full Time",
"industrial_instrument": "Wholesale Award 2010",
"pay_rate_template": "Permanent Storeworker",
"anniversary_date": "2020-07-25T00:00:00+00:00",
"salary": 50,
"salary_type": "Hour",
"pay_unit": "Hourly",
"pay_category": "Permanent Ordinary Hours",
"leave_allowance_template": "Permanent Leave Allowance",
"change_reason": "Some reason...",
"comments": "Some comments..."
}
],
"item_per_page": 20,
"page_index": 1,
"total_pages": 2,
"total_items": 2
}
}
This endpoint retrieves a list of all pay details for a specific employee.
HTTP Request
GET https://api.employmenthero.com/api/v1/organisations/:organisation_id/employees/:employee_id/pay_details
URL Parameters
Parameter | Description |
---|---|
organisation_id uuid |
The ID of the organisation to retrieve |
employee_id uuid |
The ID of the employee to retrieve |
Return
A hash with a data property that contains an array of up to the limit of pay details. Each entry in the array is a separate pay details object. If there are no more pay details, the resulting array will be empty.
Tax Declaration
The Tax Declaration Object
The Tax Declaration Object
{
"first_name": "Daniel",
"last_name": "Alves",
"tax_file_number": "000000000",
"tax_au_resident": true,
"tax_foreign_resident": false,
"working_holiday_maker": false,
"tax_free": false,
"tax_help_debt": true,
"tax_financial_supplement_debt": false
}
Attribute | Description |
---|---|
first_name string |
The first name of the employee. |
last_name string |
The last name of the employee. |
tax_file_number string |
The tax file number of the employee. |
tax_au_resident boolean |
The employee is an Australian resident for tax purposes. |
tax_foreign_resident boolean |
The employee is a foreign resident for tax purposes. |
working_holiday_maker boolean |
The employee is a working holiday maker. |
tax_free boolean |
The employee is claiming the tax free threshold from the employer. |
tax_help_debt boolean |
The employee has study and training support loan debts. |
tax_financial_supplement_debt boolean |
The employee has financial supplement debts. |
Get Tax Declaration Detail
GET
/v1/organisations/:organisation_id/employees/:employee_id/tax_declaration
curl
"https://api.employmenthero.com/api/v1/organisations/:organisation_id/employees/:employee_id/tax_declaration"
-H "Authorization: bearer AUXJ3123xyrj123fdsjkl124aAJKQ"
RESPONSE
{
"data": {
"first_name": "Daniel",
"last_name": "Alves",
"tax_file_number": "000000000",
"tax_au_resident": true,
"tax_foreign_resident": false,
"working_holiday_maker": false,
"tax_free": false,
"tax_help_debt": true,
"tax_financial_supplement_debt": false
}
}
This endpoint retrieves a tax declaration detail for a specific employee.
HTTP Request
GET https://api.employmenthero.com/api/v1/organisations/:organisation_id/employees/:employee_id/tax_declaration
URL Parameters
Parameter | Description |
---|---|
organisation_id uuid |
The ID of the organisation to retrieve |
employee_id uuid |
The ID of the employee to retrieve |
Return
A hash with a data property that contains a tax declaration detail object. If having no tax declaration, the result will be not found error.
Superannuation Detail
The Superannuation Detail Object
The Superannuation Detail Object
{
"fund_name": "SUPER Super",
"member_number": "184752",
"product_code": "294002294",
"employer_nominated_fund": true,
"fund_abn": "19905422123",
"electronic_service_address": "CLICKSUPER",
"fund_email": "some_email@email.com",
"account_name": "Daniel",
"account_bsb": "HST01100AX",
"account_number": "25767731292"
}
Attribute | Description |
---|---|
fund_name string |
The name of the employee's superannuation fund. |
member_number string |
The member number of the employee. |
product_code string |
The product code of the employee's superannuation fund. |
employer_nominated_fund boolean |
The employee has chosen the employer nominated fund. |
fund_abn string |
The ABN of the employee's superannuation fund. |
electronic_service_address string |
The Electronic Service Address of the employee's SMSF. |
fund_email string |
The email of the employee's superannuation fund. |
account_name string |
The bank account name of the employee's SMSF. |
account_bsb string |
The bank BSB of the employee's SMSF. |
account_number string |
The bank account number of the employee's SMSF. |
Get Superannuation Detail
GET
/v1/organisations/:organisation_id/employees/:employee_id/superannuation_detail
curl
"https://api.employmenthero.com/api/v1/organisations/:organisation_id/employees/:employee_id/superannuation_detail"
-H "Authorization: bearer AUXJ3123xyrj123fdsjkl124aAJKQ"
RESPONSE
{
"data": {
"fund_name": "SUPER Super",
"member_number": "184752",
"product_code": "294002294",
"employer_nominated_fund": true,
"fund_abn": "19905422123",
"electronic_service_address": "CLICKSUPER",
"fund_email": "some_email@email.com",
"account_name": "Daniel",
"account_bsb": "HST01100AX",
"account_number": "25767731292"
}
}
This endpoint retrieves a superannuation detail for a specific employee.
HTTP Request
GET https://api.employmenthero.com/api/v1/organisations/:organisation_id/employees/:employee_id/superannuation_detail
URL Parameters
Parameter | Description |
---|---|
organisation_id uuid |
The ID of the organisation to retrieve |
employee_id uuid |
The ID of the employee to retrieve |
Return
A hash with a data property that contains a superannuation detail object. If having no superannuation, the result will be not found error.
Custom Field
The Custom Field Object
The Custom Field Object
{
"id": "d8fe2759-a033-49bc-92b3-921556491b2f",
"name": "Tshirt Size",
"hint": "Unisex sizing",
"description": "Tshirt sizing for purchasing uniforms",
"type": "single_select",
"in_onboarding": false,
"required": false,
"custom_field_permissions": [
{
"id": "e4251780-7e3d-4e9a-84e7-45874e9179be",
"permission": "editable",
"role": "employee"
},
{
"id": "f7f75c55-5084-4574-9786-36e0fa4cb2d3",
"permission": "editable",
"role": "manager"
}
],
"custom_field_options": [
{
"id": "c55ee94c-72b7-4042-ac32-b3ab4ab38d12",
"value": "Large"
},
{
"id": "18e33075-7233-4c0a-9fc9-6ce48e07c807",
"value": "Medium"
},
{
"id": "ea3b8a51-3bfb-4500-bd1f-fe81620cc635",
"value": "Small"
}
]
}
Attribute | Description |
---|---|
id uuid |
The custom field ID |
name string |
The name of custom field |
hint string |
The hint of custom field |
description string |
The description of custom field |
type string |
The type of custom field, there are only 3 supported types free_text , single_select , multi_select |
in_boarding boolean |
Return true if the custom field captured during onboarding |
required boolean |
Return true if the custom field is mandatory |
custom_field_permissions array |
Lists the permissions for the custom field |
custom_field_options array |
Lists the options for the custom field when type is either single_select or multi_select |
Get All Custom Fields
GET
/v1/organisations/:organisation_id/custom_fields
curl "https://api.employmenthero.com/api/v1/organisations/:organisation_id/custom_fields"
-H "Authorization: bearer AUXJ3123xyrj123fdsjkl124aAJKQ"
RESPONSE
{
"data": {
"items": [
{
"id": "f131fbe9-eb01-4802-98d9-6df7d11a6403",
"name": "Work day preference",
"hint": "Pick the day(s) that you would prefer to be rostered on",
"description": "Tracking workday preferences to help with rostering ",
"type": "multi_select",
"in_onboarding": false,
"required": false,
"custom_field_permissions": [
{
"id": "0d61bec9-8dcf-4e54-a26f-194ec376ee70",
"permission": "editable",
"role": "employee"
},
{
"id": "9659d80b-04a7-48fb-9bd9-94e76fdf09ff",
"permission": "editable",
"role": "manager"
}
],
"custom_field_options": [
{
"id": "2c6fb761-f7b2-4ddc-a6b1-cfe734cfc54d",
"value": "Friday"
},
{
"id": "8b533545-a154-4927-a210-046872eff1d2",
"value": "Monday"
},
{
"id": "47e2c33f-42d4-4dd2-8263-daf533ecfd10",
"value": "Thursday"
},
{
"id": "3c0677ec-7c57-46c2-a584-1a1058ffe184",
"value": "Tuesday"
},
{
"id": "e66bd01e-e563-429f-b476-0956be7c5d04",
"value": "Wednesday"
}
]
},
{
"id": "d8fe2759-a033-49bc-92b3-921556491b2f",
"name": "Tshirt Size",
"hint": "Unisex sizing",
"description": "Tshirt sizing for purchasing uniforms",
"type": "single_select",
"in_onboarding": false,
"required": false,
"custom_field_permissions": [
{
"id": "e4251780-7e3d-4e9a-84e7-45874e9179be",
"permission": "editable",
"role": "employee"
},
{
"id": "f7f75c55-5084-4574-9786-36e0fa4cb2d3",
"permission": "editable",
"role": "manager"
}
],
"custom_field_options": [
{
"id": "c55ee94c-72b7-4042-ac32-b3ab4ab38d12",
"value": "Large"
},
{
"id": "18e33075-7233-4c0a-9fc9-6ce48e07c807",
"value": "Medium"
},
{
"id": "ea3b8a51-3bfb-4500-bd1f-fe81620cc635",
"value": "Small"
}
]
},
{
"id": "f6f45926-5425-417b-b010-cc840858154c",
"name": "Dietary Requirements",
"hint": "Any food restrictions",
"description": "To track dietary requirements for catering at events",
"type": "free_text",
"in_onboarding": false,
"required": false,
"custom_field_permissions": [
{
"id": "b9fe040e-0ebc-4ce6-a79c-837b80795823",
"permission": "editable",
"role": "employee"
},
{
"id": "b1522654-2ac8-4593-b51c-8bf0ce2fcfe2",
"permission": "editable",
"role": "manager"
}
]
}
],
"item_per_page": 20,
"page_index": 1,
"total_pages": 1,
"total_items": 3
}
}
This endpoint retrieves a list of all custom fields for a specific organisation.
HTTP Request
GET https://api.employmenthero.com/api/v1/organisations/:organisation_id/custom_fields
Parameter | Description |
---|---|
organisation_id uuid |
The ID of the organisation to retrieve |
Return
A hash with a data property that contains an item array of up to limit Custom Fields. Each entry in the array is a separate Custom Field object. If there are no more Custom Fields, the resulting array will be empty.
Employee Custom Field
The Employee Custom Field Object
The Employee Custom Field Object
{
"id": "f6f45926-5425-417b-b010-cc840858154c",
"value": "No eggs",
"name": "Dietary Requirements",
"description": "To track dietary requirements for catering at events",
"type": "free_text"
}
Attribute | Description |
---|---|
id uuid |
The id of the custom field |
value string OR array |
The value of the custom field filled in by your employee, it is a string when type is free_text and an array when type is single_select or multi_select |
name string |
The name of the custom field |
description string |
The description of the custom field |
type string |
The type of the custom field, there are only 3 supported types free_text , single_select , multi_select |
Get All Employee Custom Fields
GET
/v1/organisations/:organisation_id/employees/:employee_id/custom_fields
curl "https://api.employmenthero.com/api/v1/organisations/:organisation_id/employees/:employee_id/custom_fields"
-H "Authorization: bearer AUXJ3123xyrj123fdsjkl124aAJKQ"
RESPONSE
{
"data": {
"items": [
{
"id": "f6f45926-5425-417b-b010-cc840858154c",
"value": "No eggs",
"name": "Dietary Requirements",
"description": "To track dietary requirements for catering at events",
"type": "free_text"
},
{
"id": "78869738-f730-4a92-8978-dd64e5asb0be",
"value": [
{ "id": "18e33075-7233-4c0a-9fc9-6ce48e07c807", "value": "Medium" }
],
"name": "Tshirt Size",
"description": "Tshirt sizing for purchasing uniforms",
"type": "single_select"
},
{
"id": "f497b9a4-464b-4637-9c23-0e65bg9ae968",
"value": [
{ "id": "2c6fb761-f7b2-4ddc-a6b1-cfe734cfc54d", "value": "Friday" },
{ "id": "3c0677ec-7c57-46c2-a584-1a1058ffe184", "value": "Tuesday" },
{ "id": "e66bd01e-e563-429f-b476-0956be7c5d04", "value": "Wednesday" }
],
"name": "Work day preference",
"description": "Tracking workday preferences to help with rostering ",
"type": "multi_select"
}
],
"item_per_page": 20,
"page_index": 1,
"total_pages": 1,
"total_items": 3
}
}
This endpoint retrieves a list of all custom fields for a specific employee.
HTTP Request
GET https://api.employmenthero.com/api/v1/organisations/:organisation_id/employees/:employee_id/custom_fields
Parameter | Description |
---|---|
organisation_id uuid |
The ID of the organisation to retrieve |
employee_id uuid |
The ID of the employee to retrieve |
Return
A hash with a data property that contains an array of up to limit employee custom fields. Each entry in the array is a separate employee custom field object. If there are no more employee custom fields, the resulting array will be empty.
Rostered Shift
The Rostered Shift Object
The Rostered Shift Object
{
"id": "6ddbfb24-8153-42a9-9248-c41c9b6c755e",
"start_time": "2024-06-14T09:00:00.000Z",
"end_time": "2024-06-14T17:00:00.000Z",
"status": "published",
"notes": "Hello world",
"is_open_shift": false,
"location_id": "23e55174-2c7f-468d-9d11-9c387e2c3e70",
"location_name": "John Doe Hospital",
"member_id": "6664453a-c118-48f1-897e-3df62d45bd84",
"member_full_name": "John Doe",
"shift_swap_cutoff_time": null,
"type": "RosteredShift",
"work_type_id": "6ddbfb24-8153-42a9-9248-c41c9b6c755e",
"work_type_name": "Full time",
"shift_swap": {
"id": "d71690b7-381f-4d54-8bdc-e4fd9d3e3be1",
"requesting_shift_id": "4a35ccb6-8ff6-4719-b7fb-726b40467957",
"receiving_shift_id": "dbab7b49-b40b-4e0d-ae69-a5fde016c5c7",
"status": "awaiting_receiver",
"requesting_notes": null
},
"breaks": [
{
"start_time": "2024-06-14T12:00:00.000Z",
"end_time": "2024-06-14T13:00:00.000Z"
}
]
}
Attribute | Description |
---|---|
id uuid |
ID of the rostered shift |
start_time string |
Shift start time |
end_time string |
Shift end time |
status string |
Status of rostered shift |
notes string |
Notes for the shift |
is_open_shift boolean |
Whether the shift is open |
location_id uuid |
ID of the location where the shift is rostered |
location_name string |
Name of the location where the shift is rostered |
member_id uuid |
ID of the member rostered for the shift |
member_full_name string |
Full name of the member rostered for the shift |
shift_swap_cutoff_time string |
Time after which shift swap is not allowed |
type string |
Type of the shift |
work_type_id uuid |
ID of the work type of the shift |
work_type_name string |
Name of the work type of the shift |
shift_swap object |
Details of the shift swap |
breaks array |
Breaks during the shift |
List rostered shifts
GET
/v1/organisations/:organisation_id/rostered_shifts
curl "https://api.employmenthero.com/api/v1/organisations/:organisation_id/rostered_shifts"
-H "Authorization: bearer AUXJ3123xyrj123fdsjkl124aAJKQ"
RESPONSE
{
"data": {
"items": [
{
"id": "6ddbfb24-8153-42a9-9248-c41c9b6c755e",
"start_time": "2024-06-14T09:00:00.000Z",
"end_time": "2024-06-14T17:00:00.000Z",
"status": "published",
"notes": "Hello world",
"is_open_shift": false,
"type": "RosteredShift",
"work_type_id": "6ddbfb24-8153-42a9-9248-c41c9b6c755e",
"work_type_name": "Full time",
"location_id": "23e55174-2c7f-468d-9d11-9c387e2c3e70",
"location_name": "John Doe Hospital",
"member_id": "6664453a-c118-48f1-897e-3df62d45bd84",
"member_full_name": "John Doe",
"shift_swap_cutoff_time": "2024-06-14T08:00:00.000Z",
"breaks": [
{
"start_time": "2024-06-14T12:00:00.000Z",
"end_time": "2024-06-14T12:30:00.000Z"
}
],
"shift_swap": {
"id": "5b3bfb24-8153-42a9-9248-c41c9b6c755e",
"requesting_shift_id": "6ddbfb24-8153-42a9-9248-c41c9b6c755e",
"receiving_shift_id": "6ddbfb24-8153-42a9-9248-c41c9b6c755e",
"status": "rejected",
"requesting_notes": "Hello world"
}
}
],
"item_per_page": 20,
"page_index": 1,
"total_pages": 1,
"total_items": 1
}
}
List all the rostered shifts accessible by current user. The result is paginated
HTTP Request
GET https://api.employmenthero.com/api/v1/organisations/:organisation_id/rostered_shifts
Parameter | Description |
---|---|
organisation_id uuid |
ID of the organisation |
from_date string |
Start date of the range. Date equal from_date will be included |
to_date string |
End date of the range. Date equal to to_date will be included |
statuses array |
Statuses of the rostered shifts to filter by |
location_ids array |
Location IDs of the rostered shifts to filter by |
member_ids array |
Member IDs of the rostered shifts to filter by |
unassigned_shifts_only boolean |
Whether to return only unassigned shifts |
exclude_shifts_overlapping_from_date boolean |
Whether to exclude shifts that overlap with from_date. If this flag is set to true: → Retrieve the shift which start time in [from_date, to_date) Otherwise: → Retrieve the shift which start time in [from_date - 1, to_date) and end time in (from_date, to_date + 1] |
page_index number |
Page index, default is 1 |
item_per_page number |
Number of items per page, limit 100. Default is 20 |
Return
The rostered shifts current user can access
Get rostered shift by ID
GET
/v1/organisations/:organisation_id/rostered_shifts/:id
curl "https://api.employmenthero.com/api/v1/organisations/:organisation_id/rostered_shifts/:id"
-H "Authorization: bearer AUXJ3123xyrj123fdsjkl124aAJKQ"
RESPONSE
{
"id": "6ddbfb24-8153-42a9-9248-c41c9b6c755e",
"start_time": "2024-06-14T09:00:00.000Z",
"end_time": "2024-06-14T17:00:00.000Z",
"status": "published",
"notes": "Hello world",
"is_open_shift": false,
"location_id": "23e55174-2c7f-468d-9d11-9c387e2c3e70",
"location_name": "John Doe Hospital",
"member_id": "6664453a-c118-48f1-897e-3df62d45bd84",
"member_full_name": "John Doe",
"shift_swap_cutoff_time": "2024-06-14T08:00:00.000Z",
"breaks": [
{
"start_time": "2024-06-14T12:00:00.000Z",
"end_time": "2024-06-14T12:30:00.000Z"
}
],
"shift_swap": {
"id": "5b3bfb24-8153-42a9-9248-c41c9b6c755e",
"requesting_shift_id": "6ddbfb24-8153-42a9-9248-c41c9b6c755e",
"receiving_shift_id": "6ddbfb24-8153-42a9-9248-c41c9b6c755e",
"status": "rejected",
"requesting_notes": "Hello world"
}
}
Get rostered shift record using its ID
HTTP Request
GET https://api.employmenthero.com/api/v1/organisations/:organisation_id/rostered_shifts/:id
Parameter | Description |
---|---|
organisation_id uuid |
ID of the organisation |
id uuid |
ID of the rostered shift record |
Return
The rostered shift record
Unavailability
The Unavailability Object
The Unavailability Object
{
"id": "a66ac7a8-61be-4acb-9147-9908e5b12dbd",
"member_id": "6ddbfb24-8153-42a9-9248-c41c9b6c755e",
"description": "Example description",
"all_day": false,
"start_date": "2024-01-01",
"end_date": "2024-01-02",
"start_time_a_day": "09:00",
"end_time_a_day": "12:00",
"recurring_pattern": {
"recurring_type": "weekly",
"weekdays": [
"MON"
]
}
}
Attribute | Description |
---|---|
id uuid |
ID of the unavailability |
member_id uuid |
ID of the member being unavailable |
description string |
Reason for the unavailability |
all_day boolean |
true if the person is unavailable for the whole day. If false , the unavailable time range will be specified by start_time_a_day and end_time_a_day . |
start_date string |
The start date of the unavailability in ISO-8601 format (inclusive) |
end_date string |
The end date of the unavailable in ISO-8601 format (inclusive) |
start_time_a_day string |
The time within a day (in HH:mm format) when the person unavailability begins.Before this time, the person is considered available. If start_time_a_day is 06:00 , that person is considered available from 00:00 to 05:59 .If all_day is true , this field will be null . |
end_time_a_day string |
The time within a day (in HH:mm format) when the person unavailability ends.After this time, the person is considered available. If end_time_a_day is 18:00 , that person is considered available from 18:01 to 23:59 .If all_day is true , this field will be null . |
recurring_pattern object |
If null , it represents a single-day unavailability, so start_date and end_date are the same. If present, this specifies which days in the date range is unavailable.For example: With 2024-01-01 (Monday) and 2024-01-08 as start_date and end_date respectively, a recurring pattern with MON and TUE as weekdays means that member is only unavailable on 1/1/2024 (Monday) and 2/1/2024 (Tuesday). Although 3/1/2024 is in the specified date range, it does not match the recurring pattern, so it is not part of this unavailability. If we change the search range to 2024-01-03 and 2024-01-08 , we won't see the record above returned. |
List unavailabilities
GET
/v1/organisations/:organisation_id/unavailabilities
curl "https://api.employmenthero.com/api/v1/organisations/:organisation_id/unavailabilities"
-H "Authorization: bearer AUXJ3123xyrj123fdsjkl124aAJKQ"
RESPONSE
{
"data": {
"items": [
{
"id": "a66ac7a8-61be-4acb-9147-9908e5b12dbd",
"member_id": "6ddbfb24-8153-42a9-9248-c41c9b6c755e",
"description": "Example description",
"all_day": false,
"start_date": "2024-01-01",
"end_date": "2024-01-02",
"start_time_a_day": "09:00",
"end_time_a_day": "12:00",
"recurring_pattern": {
"recurring_type": "weekly",
"weekdays": [
"MON",
"TUE"
]
}
}
],
"item_per_page": 20,
"page_index": 1,
"total_items": 1,
"total_pages": 1
}
}
Get all unavailability records satisfying the provided conditions
HTTP Request
GET https://api.employmenthero.com/api/v1/organisations/:organisation_id/unavailabilities
Parameter | Description |
---|---|
organisation_id uuid |
ID of the organisation |
from_date string |
Unavailabilities must ends on or after this date to be returned. Maximum search range is 1 month. |
to_date string |
Unavailabilities must ends on or before this date to be returned. Maximum search range is 1 month. |
location_id number |
ID of the cost centre. If specified, only unavailabilities of members in this cost centre are returned. |
member_id uuid |
If set, only return unavailabilities of this member |
item_per_page number |
Number of items per result page. Must be a value in the range 1-100. |
page_index number |
The page to fetch. Page 1 is the first page. |
Return
The unavailabilities matching the specified conditions
Get unavailability by ID
GET
/v1/organisations/:organisation_id/unavailabilities/:id
curl "https://api.employmenthero.com/api/v1/organisations/:organisation_id/unavailabilities/:id"
-H "Authorization: bearer AUXJ3123xyrj123fdsjkl124aAJKQ"
RESPONSE
{
"data": {
"id": "a66ac7a8-61be-4acb-9147-9908e5b12dbd",
"member_id": "6ddbfb24-8153-42a9-9248-c41c9b6c755e",
"description": "Example description",
"all_day": false,
"start_date": "2024-01-01",
"end_date": "2024-01-02",
"start_time_a_day": "09:00",
"end_time_a_day": "12:00",
"recurring_pattern": {
"recurring_type": "weekly",
"weekdays": [
"MON",
"TUE"
]
}
}
}
Get unavailability record using its ID
HTTP Request
GET https://api.employmenthero.com/api/v1/organisations/:organisation_id/unavailabilities/:id
Parameter | Description |
---|---|
organisation_id uuid |
ID of the organisation |
id uuid |
ID of the unavailability record |
Return
The unavailability record
Errors
RESPONSE
400 Bad Request -- Your request is invalid.
401 Unauthorized -- Your API key is wrong.
403 Forbidden -- The resource requested is hidden for administrators only.
404 Not Found -- The specified resource could not be found.
406 Not Acceptable -- You requested a format that isn't json.
429 Too Many Requests -- You have exceeded the rate limit. Please try again later.
500 Internal Server Error -- We had a problem with our server. Try again later.
503 Service Unavailable -- We're temporarily offline for maintenance. Please try again later.
EmploymentHero uses conventional HTTP response codes to indicate the success or failure of an API request.
Codes in the 2xx
range indicate success.
Codes in the 4xx
range indicate an error that failed given the information provided (e.g., a required parameter was omitted, a charge failed, etc.).
Codes in the 5xx
range indicate an error with EmploymentHero's servers.
Attribute | Description |
---|---|
code string |
An HTTP status code value, without the textual description. Example values include: 400 (Bad Request), 401 (Unauthorized), and 404 (Not Found). |
error object |
A container for the error information. |
errors array |
A container for the error details. |
errors.message string |
Description of the error. Example values include Invalid argument, Login required, and Record not found. |
Pagination
RESPONSE
{
"data": {
"items": [
...
],
"item_per_page": 20,
"page_index": 1,
"total_pages": 1,
"total_items": 1
}
}
All top-level API resources support bulk fetching through list API methods.
For example, you can list organisations, teams, and employees.
These list API methods share a common structure, returning at least the following 5 parameters:
items
, page_index
, items_per_page
, total_pages
, and total_items
.
Attribute | Description |
---|---|
items Array |
A list of all resources which has been paginated |
page_index number |
The number which indicates the current page. (default: 1) |
item_per_page number |
The number which indicates the quantity of item per each page. (default: 20 and maximum: 100) |
total_pages number |
The total pages |
total_items number |
The actual total item which the system has based on your request |
Webhook
Registering a Webhook
To register a webhook, visit the My Webhooks page in the Developer Portal then click the Add Webhooks button. The webhook has 3 required fields:
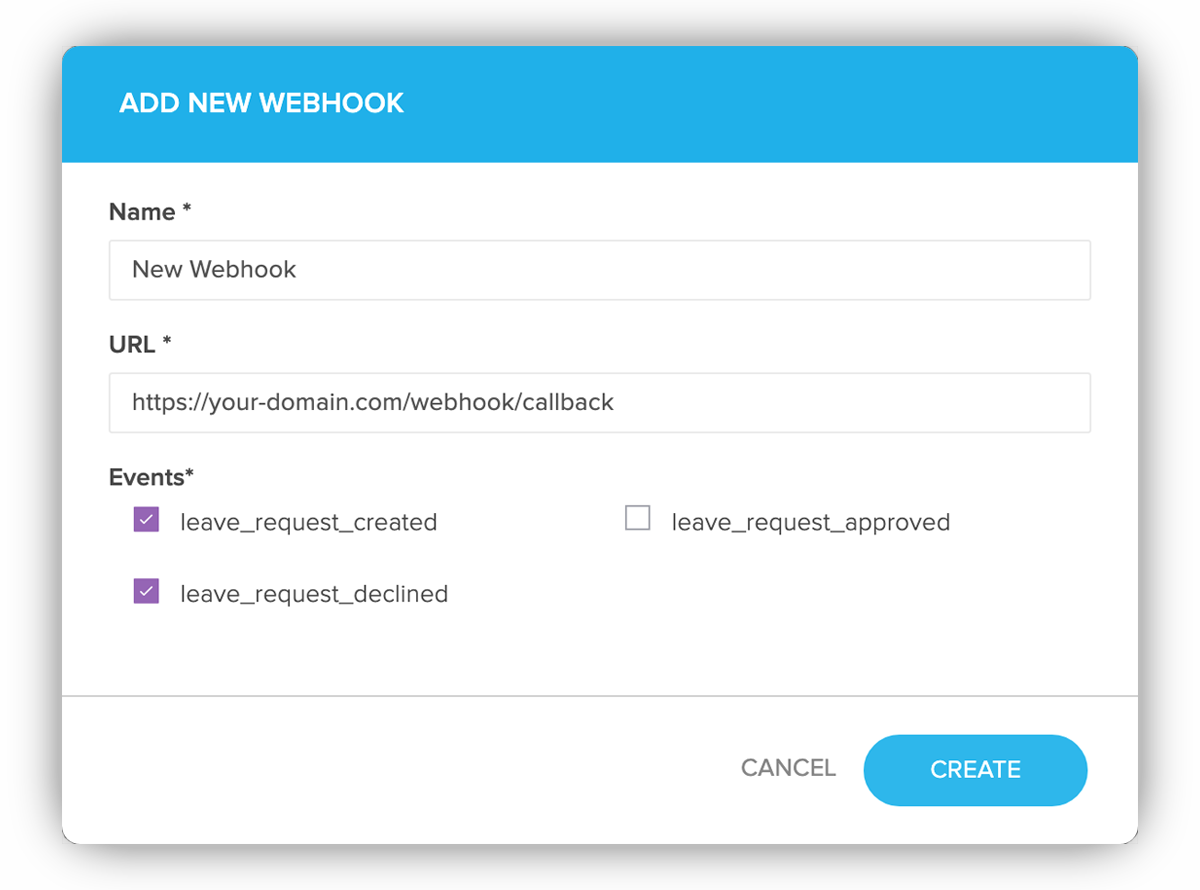
Webhook Properties
Field | Description |
---|---|
Name | Name of webhook. |
URL | The URL for the webhook event callback. Please note that for security purposes, we request that you provide HTTPS URIs. |
Events | The events that will trigger this particular webhook. |
Webhook Events
Webhook events that are currently available are:
- Employee Created
- Employee Onboarded
- Employee Updated
- Employee Offboarding
- Leave Request Created
- Leave Request Updated
- Leave Request Approved
- Leave Request Declined
- Leave Request Deleted
- Employee Bank Account Created
- Employee Bank Account Updated
- Employee Bank Account Deleted
- Employment History Created
- Employment History Updated
- Employment History Deleted
Webhook Callback
CALLBACK BODY
{
"data": {
"id": "1c8a8e41-82c8-4311-8115-07994dd28ac2",
"approved": true,
"end_date": "2020-02-04",
"start_date": "2020-02-04",
"employee_id": "ba967883-a3db-44a0-b807-bfac746599f7"
},
"event": "leave_request_approved"
}
When an event occurs, it will trigger an HTTP request to the URL configured for the webhook. If the callback URL does not respond with a successful result (i.e. HTTP 200), it will be retried for a maximum of 3 times. If the callback URL still responds unsuccessfully, the webhook event will be marked as failed.
Attribute | Description |
---|---|
event string |
The type of webhook event |
data object |
The payload of webhook event |
To see the history of webhook events, visit the Webhook History page or Webhook > Event History
on the navigation bar from the Developer Portal.
Employee Data
CALLBACK BODY
{
"data": {
"id": "f5e41ec5-b85d-486a-9bd0-ef9b04284c6e",
"gender": "male",
"country": "AU",
"known_as": "New employee",
"job_title": "Tii",
"last_name": "Please",
"first_name": "Yes",
"middle_name": "Midle Name",
"avatar_url": "/avatar.svg",
"start_date": "2020-02-27",
"external_id": "123-123-123",
"nationality": "Australia",
"account_email": "abc@def.com",
"date_of_birth": "1980-2-2",
"personal_mobile_number": "+2222222222"
},
"event": "employee_created"
}
Attribute | Description |
---|---|
id uuid |
The employee id |
gender string |
The gender of employee |
country string |
The country of employee (i.e AU, VI, US) |
known_as string |
The preferred name of employee |
job_title string |
The Job title of employee |
last_name string |
The last name of employee |
first_name string |
The first name of employee |
middle_name string |
The middle name of employee |
avatar_url string |
The avatar url of employee |
start_date string |
The start date of employee |
external_id string |
The external id of employee (Id of payroll system) |
nationality string |
The nationality of employee (i.e Australia, United State) |
account_email string |
The email of employee account |
date_of_birth string |
The birthday of employee |
Leave Request Data
CALLBACK BODY
{
"data": {
"id": "1c8a8e41-82c8-4311-8115-07994dd28ac2",
"approved": true,
"end_date": "2020-02-04",
"start_date": "2020-02-04",
"employee_id": "ba967883-a3db-44a0-b807-bfac746599f7"
},
"event": "leave_request_approved"
}
Attribute | Description |
---|---|
id uuid |
The leave request id |
approved boolean |
The approve status of leave request |
start_date date |
The start date of leave request |
end_date date |
The end date of leave request |
employee_id uuid |
The employee id of leave request |
Bank Account Data
CALLBACK BODY
{
"data": {
"id": "78bc2d65-bf9e-434f-8b78-2367f56878ad",
"bsb": "123123",
"account_name": "mew123",
"account_number": "123123",
"primary_account": false
},
"event": "bank_account_created"
}
Attribute | Description |
---|---|
id uuid |
The bank account id |
bsb string |
The bsb number of bank account |
account_name string |
The account name of bank account |
account_number string |
The account number of bank account |
primary_account boolean |
Determine bank account is primary or not |
Employment History Data
CALLBACK BODY
{
"data": {
"id": "4f6c7312-67ab-4775-ab2e-a3761ff56385",
"title": "Software Developer",
"disabled": false,
"end_date": "2021-12-09T00:00:00.000+00:00",
"in_review": false,
"member_id": "e826e79d-20d4-4b04-add2-8fa3c16cb7d8",
"start_date": "2021-02-17T00:00:00.000+00:00",
"declined_at": null,
"employment_type": null,
"organisation_id": "bdfcb02b-fcc3-4f09-8636-c06c14345b86"
},
"event": "employment_history_created"
}
Attribute | Description |
---|---|
id uuid |
The EmploymentHistory id |
title string |
The Job title |
end_date string |
The end date of EmploymentHistory |
start_date string |
The start date of EmploymentHistory |
in_review boolean |
Determine employment history is being reviewed or not |
disabled boolean |
Determine employment history is disabled or not |
member_id string |
The Member Id of EmploymentHistory |
organisation_id string |
The Organisation Id of EmploymentHistory |
employment_type string |
The Employment Type of EmploymentHistory (Full-time, Part-time...) |
declined_at string |
The time when EmploymentHistory is declined |